下面是一个使用冒泡排序算法对整数数组进行排序的Java代码示例:
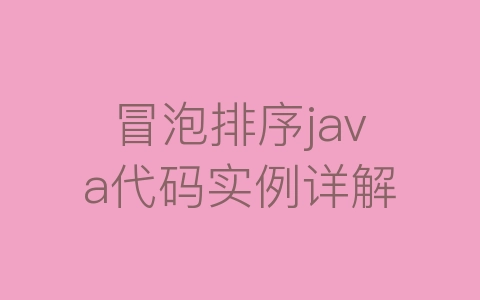
javaCopy codepublic class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// 交换arr[j]和arr[j + 1]的位置
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
public static void main(String[] args) {
int[] arr = {5, 2, 8, 12, 1, 6};
bubbleSort(arr);
System.out.println("排序结果:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
这段代码定义了一个名为BubbleSort
的类,其中包含了一个bubbleSort
方法用于执行冒泡排序算法。在main
方法中,创建一个整数数组并调用bubbleSort
方法对数组进行排序,然后打印排序结果。输出结果将会是:1 2 5 6 8 12
,即按升序排列的原始数组。